Are you looking for an answer to the topic “python multithreading return value“? We answer all your questions at the website barkmanoil.com in category: Newly updated financial and investment news for you. You will find the answer right below.
Keep Reading

How do you return a value from a thread in Python?
format(bar) return ‘foo’ + baz from multiprocessing. pool import ThreadPool pool = ThreadPool(processes=1) async_result = pool. apply_async(foo, (‘world’, ‘foo’)) # tuple of args for foo # do some other stuff in the main process return_val = async_result. get() # get the return value from your function.
How do you return a thread value?
tl;dr a thread cannot return a value (at least not without a callback mechanism). You should reference a thread like an ordinary class and ask for the value.
how to return value from function when doing threading in python using queue
Images related to the topichow to return value from function when doing threading in python using queue

Is multithreading useless in Python?
So Multithreading is 10 seconds slower than Serial on cpu heavy tasks, even with 4 threads on a 4 cores machine. Actually the difference is negligible because it’s 10 seconds on a 27 minutes job (0.6% slower), but still, it shows that multithreading is useless in this case.
How do you return a value from a function in Python?
A return statement is used to end the execution of the function call and “returns” the result (value of the expression following the return keyword) to the caller. The statements after the return statements are not executed. If the return statement is without any expression, then the special value None is returned.
How do you run multiple items at once in Python?
- def a():
- print(“Function a is running at time: ” + str(int(time. time())) + ” seconds.”)
- def b():
- print(“Function b is running at time: ” + str(int(time. time())) + ” seconds.”)
- threading. Thread(target=a). start()
- threading. Thread(target=b). start()
What is the method to retrieve the list of all active threads?
In the java console, hit Ctrl-Break. It will list all threads plus some information about the heap.
Can threads return values?
When creating a multithreaded program, we often implement the runnable interface. Runnable does not have a return value. To get the return value, Java 5 provides a new interface Callable, which can get the return value in the thread.
See some more details on the topic python multithreading return value here:
Get return value from a threaded function – Python Forum
Threads never “return” values because there is no place that they can return them to. But you can use shared resources, which you have to make …
Return một giá trị trong threading python? – Dạy Nhau Học
Còn ko bạn cứ search: thread return value python mình thấy cũng có vài cách dùng Queue. 4 Likes. thanhtrung2314 (Trung Nguyen) August 5, 2017, 3 …
how to get return value from thread in python Code Example
import threading import queue my_queue = queue.Queue() def storeInQueue(f): def wrapper(*args): my_queue.put(f(*args)) return wrapper …
Using Python Threading and Returning Multiple Results …
Returning values from threads is not possible and, as such, in this example we pass in a globally accessible (to all threads) “results” array with the index …
Can thread run method return?
tl;dr a thread cannot return a value (at least not without a callback mechanism). You should reference a thread like an ordinary class and ask for the value.
How do I return a value from runnable?
The run method of Runnable has return type void and cannot return a value.
Does multithreading improve performance Python?
^so at any time point of time, only one thread will be serving content to client… so no point of actually using multithreading to improve performance. right? Short answer: If your code is mostly waiting (for responses from the network for example), multithreading will work just fine to parallelize that waiting.
Is Python truly multithreaded?
Python is NOT a single-threaded language. Python processes typically use a single thread because of the GIL. Despite the GIL, libraries that perform computationally heavy tasks like numpy, scipy and pytorch utilise C-based implementations under the hood, allowing the use of multiple cores.
Is multithreading faster than multiprocessing?
Threads are faster to start than processes and also faster in task-switching. All Threads share a process memory pool that is very beneficial. Takes lesser time to create a new thread in the existing process than a new process.
Python Multiprocessing Guide: Returning Output From A Process
Images related to the topicPython Multiprocessing Guide: Returning Output From A Process

How would you return a value from a function?
To return a value from a function, you must include a return statement, followed by the value to be returned, before the function’s end statement. If you do not include a return statement or if you do not specify a value after the keyword return, the value returned by the function is unpredictable.
How can I return multiple values from a function?
We can return more than one values from a function by using the method called “call by address”, or “call by reference”. In the invoker function, we will use two variables to store the results, and the function will take pointer type data. So we have to pass the address of the data.
What is Setattr () used for in Python?
Python setattr() function is used to assign a new value to the attribute of an object/instance. Setattr in python sets a new specified value argument to the specified attribute name of a class/function’s defined object.
How do you use multiple threads in Python?
To use multithreading, we need to import the threading module in Python Program. A start() method is used to initiate the activity of a thread. And it calls only once for each thread so that the execution of the thread can begin.
How do I start multiple threads at the same time in Python?
- import multiprocessing.
-
- def worker(num):
- “”” Worker procedure.
- “””
- print(‘Worker:’, str(num))
-
- # Mind the “if” instruction!
How do I run a parallel thread in Python?
In fact, a Python process cannot run threads in parallel but it can run them concurrently through context switching during I/O bound operations. This limitation is actually enforced by GIL. The Python Global Interpreter Lock (GIL) prevents threads within the same process to be executed at the same time.
What is the method to retrieve the list of all active threads Python?
threading. enumerate() returns a list of all Thread objects currently alive. The list includes daemonic threads, dummy thread objects created by current_thread(), and the main thread.
How do you check the status of a thread in Python?
is_alive() method is an inbuilt method of the Thread class of the threading module in Python. It uses a Thread object, and checks whether that thread is alive or not, ie, it is still running or not. This method returns True before the run() starts until just after the run() method is executed.
Which method is used to identify a thread in Python?
The isAlive() method tests whether the thread is alive or not at any given point of time. Other threads can call a thread’s join() method to join any thread. This blocks the calling thread until the thread whose join() method is called is terminated.
Can you pass arguments to a thread?
You can only pass a single argument to the function that you are calling in the new thread. Create a struct to hold both of the values and send the address of the struct.
Python Threading Tutorial: Run Code Concurrently Using the Threading Module
Images related to the topicPython Threading Tutorial: Run Code Concurrently Using the Threading Module
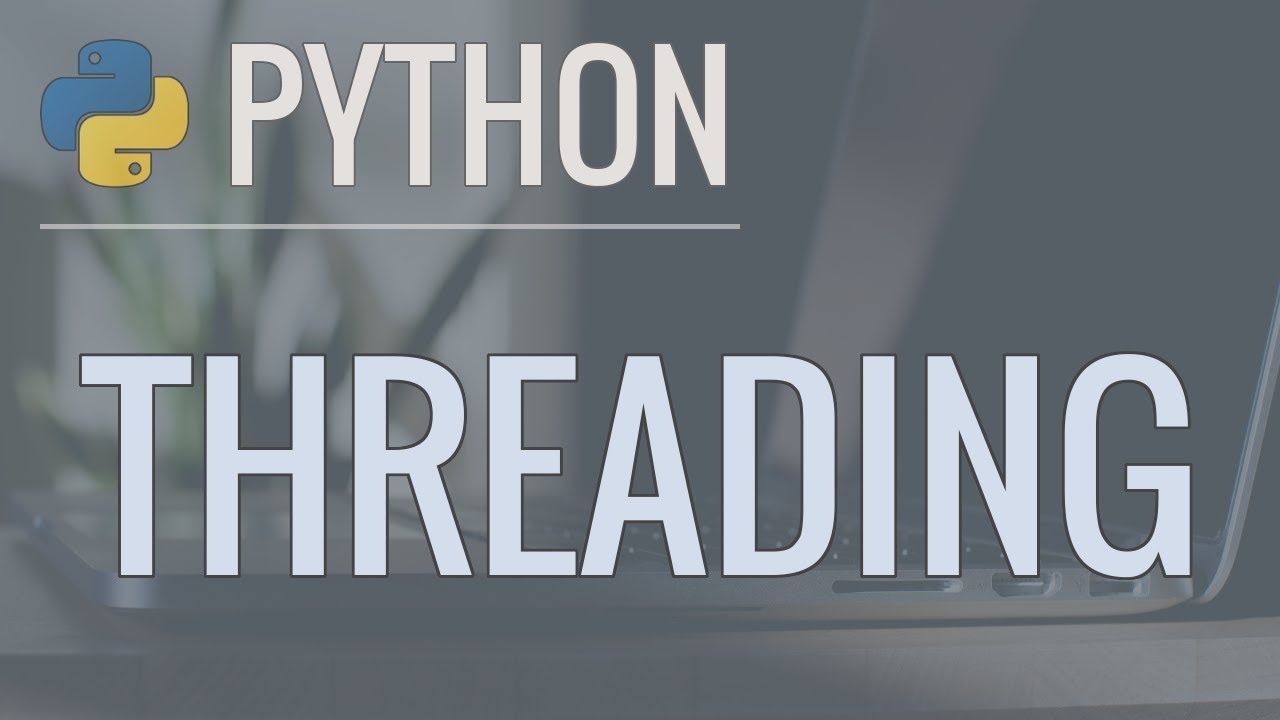
Does Pthread_exit return?
RETURN VALUE
The pthread_exit() function cannot return to its caller.
What does Pthread_self return?
The pthread_self() function returns the ID of the thread in which it is invoked.
Related searches to python multithreading return value
- python multiprocessing target return value
- threading lock python
- race condition python
- python multithreading for loop with return value
- Threading lock Python
- python multithreading function return value
- Get return value thread C
- Java thread return value
- thread return value python
- java thread return value
- Get return value from multiprocessing python
- python return multiple value
- python return thread value
- Thread return value python
- threading python
- get return value from multiprocessing python
- how to end thread python
- python multi thread get return value
- get return value thread c
- Threading Python
Information related to the topic python multithreading return value
Here are the search results of the thread python multithreading return value from Bing. You can read more if you want.
You have just come across an article on the topic python multithreading return value. If you found this article useful, please share it. Thank you very much.