Are you looking for an answer to the topic “python inner class inheritance“? We answer all your questions at the website barkmanoil.com in category: Newly updated financial and investment news for you. You will find the answer right below.
Inner classes
A inner class declared in the same outer class (or in its descendant) can inherit another inner class.No, an inner class can’t inherit (not extend) its outer class because the outer class is not fully defined while defining the inner class.A parent class can have one or more inner class but generally inner classes are avoided. We can make our code even more object oriented by using inner class. A single object of the class can hold multiple sub-objects. We can use multiple sub-objects to give a good structure to our program.
- It represents real-world relationships well.
- It provides reusability of a code. We don’t have to write the same code again and again. …
- It is transitive in nature, which means that if class B inherits from another class A, then all the subclasses of B would automatically inherit from class A.
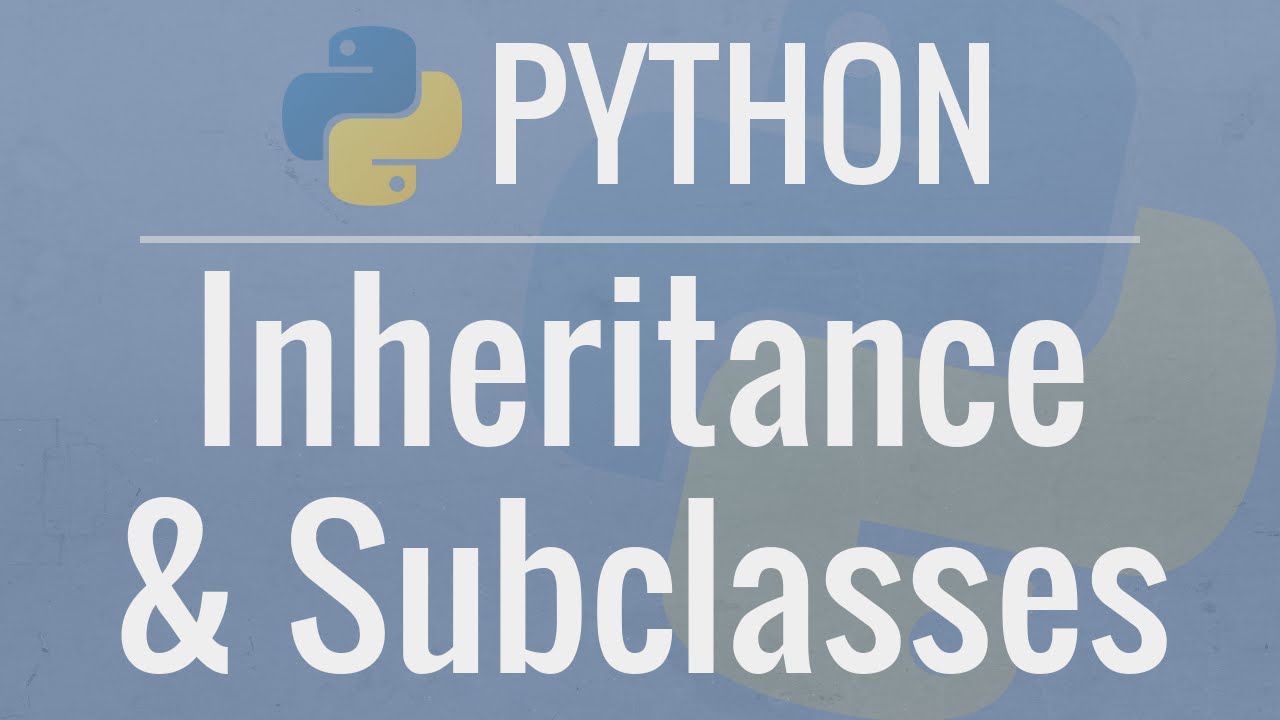
Can inner classes be inherited?
Inner classes
A inner class declared in the same outer class (or in its descendant) can inherit another inner class.
Can inner class inherit outer class in Python?
No, an inner class can’t inherit (not extend) its outer class because the outer class is not fully defined while defining the inner class.
Python OOP Tutorial 4: Inheritance – Creating Subclasses
Images related to the topicPython OOP Tutorial 4: Inheritance – Creating Subclasses
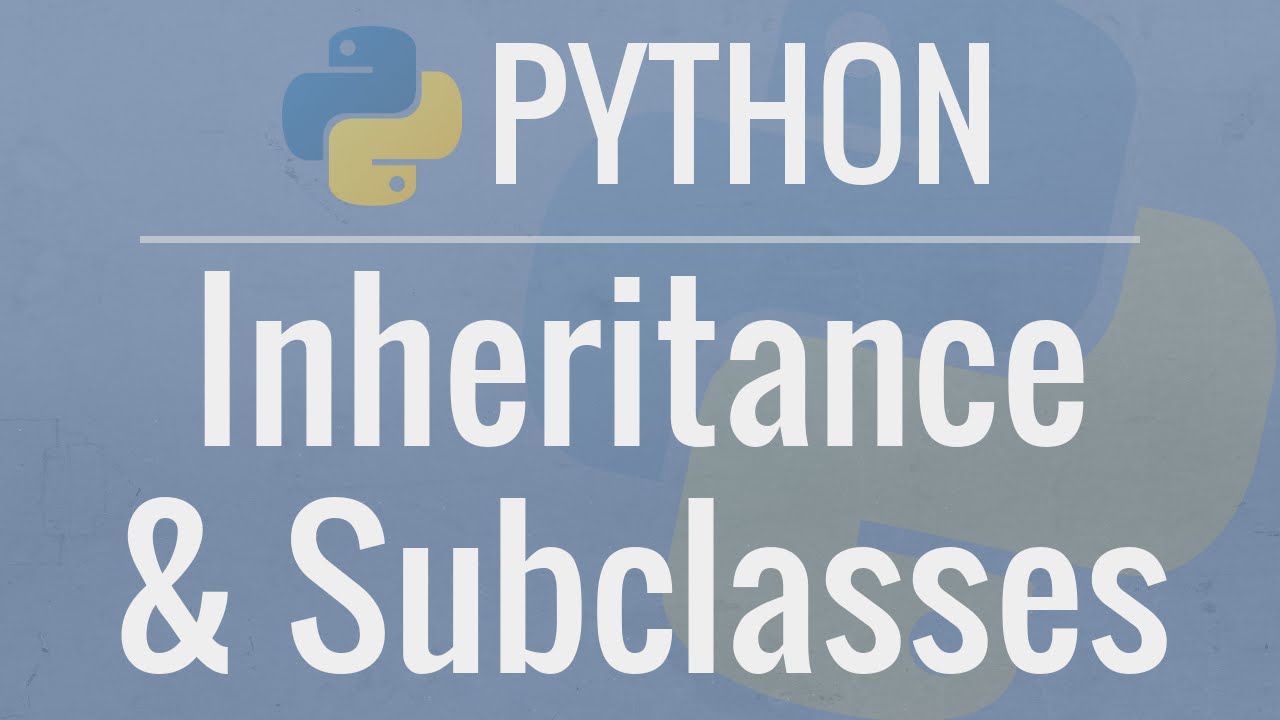
Why inner classes are avoided in Python?
A parent class can have one or more inner class but generally inner classes are avoided. We can make our code even more object oriented by using inner class. A single object of the class can hold multiple sub-objects. We can use multiple sub-objects to give a good structure to our program.
Can we create class inside class in Python?
You can have more than one inner class in a class. As we defined earlier, it’s easy to implement multiple inner classes. class Outer: “””Outer Class””” def __init__(self): ## Instantiating the ‘Inner’ class self.
How do I inherit a nested class?
A nested class may inherit from private members of its enclosing class. The following example demonstrates this: class A { private: class B { }; B *z; class C : private B { private: B y; // A::B y2; C *x; // A::C *x2; }; }; The nested class A::C inherits from A::B .
What is an inner class and how it is different from an inheritance?
Ans. Inner Class is a class that is nested within another class whereas sub class is a class that extends or inherit another class.
What is the __ init __ method?
The __init__ method is similar to constructors in C++ and Java . Constructors are used to initialize the object’s state. The task of constructors is to initialize(assign values) to the data members of the class when an object of class is created.
See some more details on the topic python inner class inheritance here:
Understanding inheritance in Python inner class – CodeSpeedy
Inheritance is a concept in which one class can derive the methods and variables from another class. Just like a child inherits some traits from his parents. In …
[Solved] Inheritance and inner classes in Python? – Local Coder
Inheritance is a per-class thing. In your code class B inherits from class A , but just because both of them have inner class Foo doesn’t tell us anything …
Python nested class inheritance – 免费编程教程
Python Inheritance Inheritance allows us to define a class that inherits all the methods and properties from another class. Parent class is the class being …
Python Nested & Inner Classes | DataCamp
In this basic Python tutorial, you’ll learn about why and when you should use inner classes. You have probably heard of the nested functions in Python. If you …
What class can an inner class extend?
Inner class can extend it’s outer class. But, it does not serve any meaning. Because, even the private members of outer class are available inside the inner class. Even though, When an inner class extends its outer class, only fields and methods are inherited but not inner class itself.
Is there multiple inheritance in Python?
A class can be derived from more than one base class in Python, similar to C++. This is called multiple inheritance. In multiple inheritance, the features of all the base classes are inherited into the derived class.
What is __ init __ in Python?
The __init__ method is the Python equivalent of the C++ constructor in an object-oriented approach. The __init__ function is called every time an object is created from a class. The __init__ method lets the class initialize the object’s attributes and serves no other purpose. It is only used within classes.
Is nested classes bad?
They’re not “bad” as such. They can be subject to abuse (inner classes of inner classes, for example). As soon as my inner class spans more than a few lines, I prefer to extract it into its own class. It aids readability, and testing in some instances.
Does Python support nested classes?
Let us try to get an overview of the nested class in python. A class defined in another class is known as Nested class. If an object is created using nested class then the object can also be created using the Parent class. Moreover, a parent class can have multiple nested class in it.
9. Python Classes and Inheritance
Images related to the topic9. Python Classes and Inheritance

How do you call a class within a class in Python?
Call method from another class in a different class in Python. we can call the method of another class by using their class name and function with dot operator. then we can call method_A from class B by following way: class A: method_A(self): {} class B: method_B(self): A.
Can you have a class inside a class?
A nested class is a member of its enclosing class. Non-static nested classes (inner classes) have access to other members of the enclosing class, even if they are declared private. Static nested classes do not have access to other members of the enclosing class.
How do you access a class within a class?
They are accessed using the enclosing class name. To instantiate an inner class, you must first instantiate the outer class. Then, create the inner object within the outer object with this syntax: OuterClass.
Can you extend a static class?
Just like static members, a static nested class does not have access to the instance variables and methods of the outer class. You can extend static inner class with another inner class.
How do you access the outer class variable from the inner class in Python?
- class Outer(object):
- def __init__(self):
- self. outer_var = 1.
- def get_inner_object(self):
- return self. Inner(self) included to access inner class.
- class Inner(object):
- def __init__(self, outer):
- self. outer = outer.
What class must an inner class extend Mcq?
It must extend the enclosing class.
What is the difference between an inner class and a sub class in Python?
inner classes are in the same file, whereas subclasses can be in another file, maybe in another package. You cannot get an instance of an inner class without an instance of the class that contains it. inner classes have the methods they want, whereas subclasses have the methods of their parent class.
What is the difference between inner class and nested class?
In Java programming, nested and inner classes often go hand in hand. A class that is defined within another class is called a nested class. An inner class, on the other hand, is a non-static type, a particular specimen of a nested class.
What is the difference between nested class and derived class?
If a class is nested in the public section of a class, it is visible outside the surrounding class. If it is nested in the protected section it is visible in derived classes, if it is nested in the private section, it is only visible for the members of the outer class.
Is __ init __ necessary?
No, it is not necessary but it helps in so many ways. people from Java or OOPS background understand better. For every class instance, there is an object chaining that needs to complete when we instantiate any class by creating an object.
Python Tutorial – Inner Classes
Images related to the topicPython Tutorial – Inner Classes
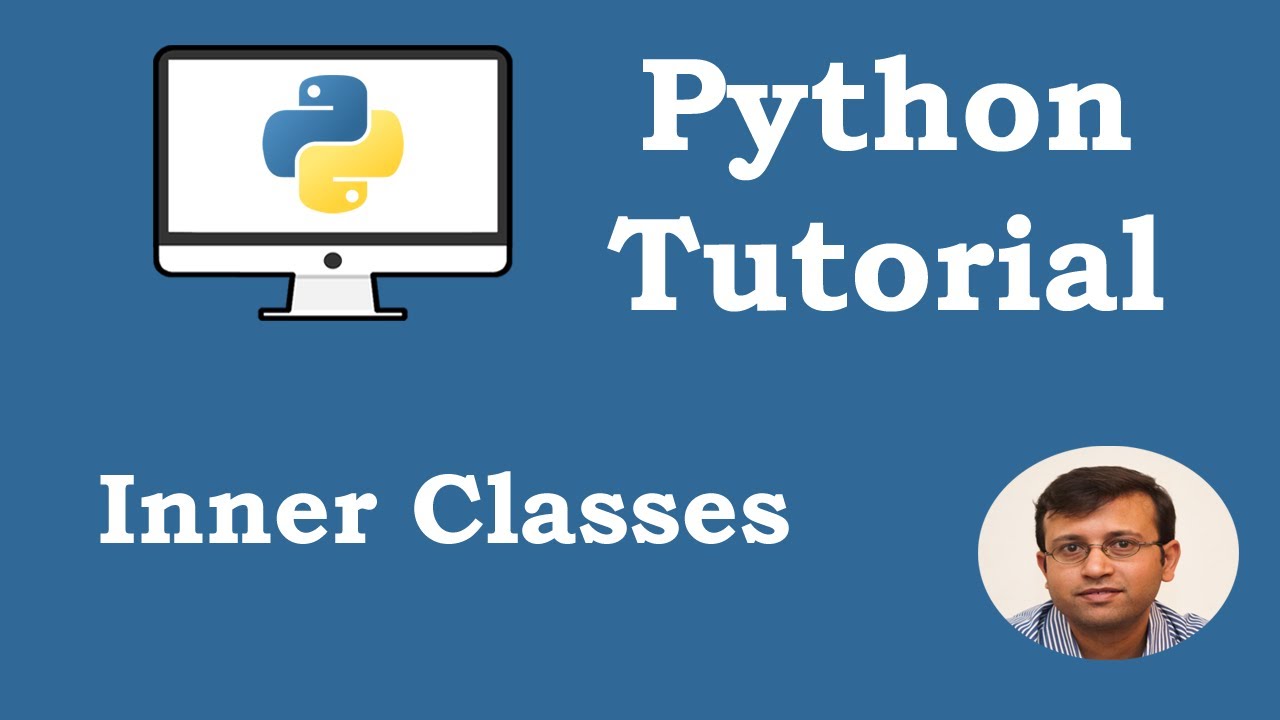
What does super () __ Init__ do?
Understanding Python super() with __init__() methods
It is known as a constructor in Object-Oriented terminology. This method when called, allows the class to initialize the attributes of the class. The super() function allows us to avoid using the base class name explicitly.
What does __ init __( self mean?
__init__ is the constructor for a class. The self parameter refers to the instance of the object (like this in C++). class Point: def __init__(self, x, y): self._x = x self._y = y.
Related searches to python inner class inheritance
- python inherit class method
- python example class inheritance
- how to create an instance of a class in another class in python
- python class inheritance
- python get class inheritance
- python inner class vs inheritance
- python nested class good practice
- inheritance in python examples
- python class class
- python subclass vs inheritance
- python inherit attributes from parent class
- python inner class inherit outer class
- python class inheritance super example
- nested class inheritance java
- python class inherit list
- python class inheritance function
- python define class inheritance
- python inner class access outer class
- import inner class python
Information related to the topic python inner class inheritance
Here are the search results of the thread python inner class inheritance from Bing. You can read more if you want.
You have just come across an article on the topic python inner class inheritance. If you found this article useful, please share it. Thank you very much.