How do I delete all unpushed commits?
You can use the git reset command to achieve this. It’s important to note that this command permanently removes the commits from your local history, so be sure to back up your work before proceeding.
To use git reset, you’ll need the commit hash of the commit you want to reset to. This is like a unique identifier for that specific commit. You can find the commit hash by using git log to see your commit history.
Once you have the commit hash, you can use this command:
git reset –hard
This will reset your current branch to the specified commit, discarding all commits that were made after that point.
Now let’s dig a little deeper into what this means:
git reset – The command itself, which allows us to move back to a previous point in your history.
–hard – This is a very important option. It tells git to completely discard any changes made since the specified commit. This includes both the changes in the files and the commit messages themselves. Think of it as wiping your slate clean to that point.
Important Considerations:
Safety First: Always back up your work before using git reset. It’s a powerful command, and you don’t want to accidentally lose your work.
Force Push: Be careful about pushing changes after a git reset to a remote repository. This might overwrite the history of the repository, potentially causing issues for others who are collaborating with you. If you’re working on a branch that hasn’t been shared yet, you’re probably fine. But for shared branches, it’s crucial to avoid pushing directly and to work with your team to coordinate changes.
Using git reset is a powerful tool to help you manage your local Git history. Remember to proceed with caution, and always make a backup of your work before using this command!
How do I clear all commits in git?
git reset is a powerful command that lets you rewind your repository to a specific commit. It’s a handy tool for fixing mistakes or cleaning up your commit history.
To remove the last commit, you can use git reset –hard HEAD^. This command moves your HEAD pointer to the commit before the current one. If you want to remove more than one commit, you can use git reset –hard HEAD~2 to remove the last two commits. Simply increase the number after the ~ to remove more commits.
Important Note: Remember, git reset –hard is a destructive operation. If you’re unsure, it’s best to create a backup of your repository before using it.
Here’s a more detailed explanation:
git reset is a command that moves your HEAD pointer, which is like a marker that points to the current state of your branch.
–hard tells git reset to also modify your working directory and staging area to match the state of the commit you’re resetting to.
HEAD^ refers to the parent commit of the current commit (the commit just before the current one).
HEAD~2 refers to the commit that is two commits behind the current commit (the commit two commits before the current one).
The process of using git reset –hard is like rewinding a video tape: You are rolling back to a specific point in the history of your project, and all changes made after that point are discarded.
A few more things to consider about using git reset –hard:
After using git reset –hard, you’ll lose any uncommitted changes. Make sure you’ve saved any important changes before using this command.
Using git reset –hard can be risky if you’re working on a shared repository. If other people are working on the same repository, they might have changes that you’ll overwrite with git reset –hard.
Once you use git reset –hard, you can’t easily undo it. You might be able to recover some of the lost commits using a technique called git reflog, but it’s not always guaranteed.
Always use git reset –hard with caution, and make sure you understand the risks before using it.
How do I delete a local commit?
Now you are ready to delete the local commit! You’re going to edit the .git/COMMIT_EDITMSG file. Inside this file, you’ll find a list of commits. To delete a commit, you can either remove its line or change the word pick to drop. This will tell Git to remove the commit when you rebase your branch.
If you change the word pick to drop, Git will not delete the commit, but it will simply skip it when it rebases your branch. This is useful if you want to keep the commit but not include it in your current branch.
Once you have finished editing the file, save it and exit. Then, run the command git rebase –continue to continue the rebase. Git will then apply the remaining commits in the list, skipping or removing the commit you marked for deletion or dropping.
You can also edit the .git/COMMIT_EDITMSG file to remove or change the commit message. This is useful if you made a mistake in the commit message. Once you have finished editing the file, save it and exit. Then, run the command git rebase –continue to continue the rebase. Git will then apply the remaining commits in the list, including the commit with the updated message.
Keep in mind that when you rebase your branch, you are rewriting its history. This means that you should only rebase branches that are not shared with other developers. If you are working on a shared branch, you should use git revert to undo a commit.
How to remove all files not in git?
git clean -f -x is a powerful command that will delete all untracked files in your repository. It removes files that are not in your .gitignore file, as well as files that are ignored by .gitignore.
Let’s break down the git clean command options:
-f (force): This option tells Git to go ahead and remove files without asking for confirmation. Be careful using this option if you’re not sure what files you are deleting.
-x (exclude ignored files): This option will include files that are ignored by your .gitignore file in the deletion.
Before you use git clean -f -x, it’s always a good idea to double-check that you don’t need any of the files you are about to delete. You can use git status to see a list of all untracked files in your repository.
Here’s an example:
“`bash
git clean -f -x
“`
This command will delete all untracked files in your repository, including files that are ignored by your .gitignore file.
Remember: If you’re not sure if you need to remove a file, it’s always best to err on the side of caution and don’t delete it. You can always remove it later if you’re sure you don’t need it anymore.
How to remove all uncommitted files in git?
Git checkout is your friend for discarding changes to a single file. If you want to revert changes to a specific file, you can use the command git checkout —
If you’re feeling bolder and want to wipe the slate clean for your entire repository, Git reset –hard is your go-to command. This powerful command discards all uncommitted changes, bringing your repository back to the state of the last commit. Just remember, this is a destructive action, so be sure to back up any important files before executing this command.
Here’s a breakdown of how git reset –hard works:
– Resets the HEAD pointer: The HEAD pointer is like a marker in your repository that points to the current commit. git reset –hard moves the HEAD pointer back to a specific commit, typically the last commit.
– Rewrites the working directory: This means it updates the files in your working directory to match the state of the commit pointed to by the HEAD.
– Clears the staging area: This area, also known as the index, is where you stage changes before committing them. git reset –hard empties the staging area, ensuring there are no changes waiting to be committed.
Keep in mind, git reset –hard will permanently remove any uncommitted changes, so always double-check that you’re ready to lose these changes before executing the command. If you’re unsure, it’s best to back up your work or consider using git stash as a safer alternative to temporarily store changes you might need later.
How do I reset all previous commits in git?
Think of it like hitting the “undo” button on a massive scale. The –hard flag lets you bring your Git repository back to an earlier state, as if all the subsequent commits never happened. It essentially rewrites history by discarding everything that came after the selected commit. This includes all changes to files, branches, and even the entire repository. It’s a drastic action, and you should only use it if you’re absolutely sure you want to get rid of everything that happened since that point in time.
Here’s a breakdown of how it works:
1. Identify the commit: First, you need to know the exact commit you want to revert to. You can find the commit ID using `git log`.
2. Use the `git reset` command: You’ll then use the command `git reset –hard
3. Caution: Before you execute this command, it’s crucial to back up your work. This is a permanent change that can’t be undone! If you’re not certain about using –hard, consider using `git revert` instead. Revert creates a new commit that undoes the changes of the previous commit, preserving your history.
In essence, using –hard is like taking a time machine back to a specific point in your Git repository and erasing everything that happened afterward. While it’s a powerful tool, it requires careful consideration and understanding of its implications. Always remember to create backups before performing such drastic actions.
See more here: How To Remove All Not Commited Files In Git? | Git Remove All Unpushed Commits
How to remove an unpushed commit from a git repository?
We’ll use the –soft option with git reset to remove the unwanted commit while preserving your local changes. To target a specific commit, you can use the HEAD~1 notation, which removes only the last commit.
Let’s break down how this works:
git reset is a powerful command used to move the HEAD pointer (the current branch) to a different point in the commit history.
–soft means that the changes from the removed commit will remain in your working directory and staging area. You’ll need to commit them again if you want to keep them.
HEAD~1 refers to the commit immediately before the current HEAD. It’s a shorthand way of specifying the commit you want to reset to.
Think of it like taking a step back in time. You’re essentially rewinding your branch to a point before the unwanted commit, but your changes remain untouched. This allows you to fix mistakes, try out different approaches, or simply clean up your commit history.
Here’s how you would apply this:
1. Identify the commit: Make sure you know the commit you want to remove. Use the git log command to review your commit history.
2. Run the command: Execute the command git reset –soft HEAD~1. This will remove the last commit. You can modify HEAD~1 to target a different commit if needed.
3. Confirm the changes: Use git log to verify the commit was removed.
4. Commit again: If you want to keep the changes, commit them again using git add followed by git commit.
Important Note: This only affects your local repository. If you’ve already pushed your commits to a remote repository, you’ll need to use a more advanced technique like git revert or git rebase to remove the commit from the shared history.
How do I remove unpushed commits from my repository history?
To do this, identify the commit you want to reset to. You can use git log to see a list of your commits. Once you find the commit, use this command to reset your branch to that commit:
git reset –hard
Important: This will also remove all the changes introduced by the commits you’re discarding. It’s like rewinding your repository to that point in time, so make sure you understand the implications before using this command.
Remember that if you’ve already pushed these commits to a remote repository, resetting your branch won’t change the history there. You might need to use `git push -f` to force push your local changes to the remote, but this can cause problems if others have already pulled your changes. Always communicate with your team before force pushing to a remote repository.
Think of it like this: git reset –hard is a powerful tool for correcting mistakes, but it’s like using a heavy-duty eraser. Use it carefully! If you’re not sure what you’re doing, ask for help or make a backup of your repository first.
Is the unpushed commit still present in Git?
Let’s break down what happened:
git reset –soft HEAD~1: This command moved your HEAD back one commit, effectively undoing the latest commit. But, as the name “soft” implies, it only undid the commit, not the changes themselves.
git reset –hard HEAD~1: This command is more drastic. It also moves your HEAD back one commit, but it completely discards the changes made in that commit. Think of it as a rewind button that goes back in time and erases any changes made in the last commit.
So, if you want to keep your changes, use the git reset –soft HEAD~1 command. You can then commit the changes again with a new message if you want to update the commit history. If you don’t want to keep the changes, use git reset –hard HEAD~1 to completely discard them.
Let’s say you have a file named ‘my_file.txt’ and you’ve made some changes to it. You’ve staged those changes, but then you realize you made a mistake. You want to undo the changes and start fresh. Here’s what you can do:
1. git reset –soft HEAD~1: This undoes the commit you made, but your changes are still there in the staging area.
2. git checkout my_file.txt: This discards the changes you made to the file and brings back the previous version. Now you can make the correct changes and start over.
3. git add my_file.txt: Stage the correct changes for the file.
4. git commit -m “Corrected changes to my_file.txt”: Commit your changes with a new commit message.
Remember, git reset –hard should be used with caution as it permanently deletes changes. Make sure you understand what you’re doing before using this command!
How do I delete all unpushed commits?
Here’s how to do it:
1. `git push origin HEAD:master –force` – This command is the most direct way to force push your local branch onto the remote. This will overwrite anything on the remote branch with the contents of your local branch.
2. `git push -f origin master` – This command achieves the same result as the previous command. The `-f` option stands for force, and it ensures that your local branch overwrites any existing content on the remote branch.
It’s important to understand the implications of force pushing. It overwrites the entire remote branch, meaning any work done on the remote by other collaborators will be lost.
Here’s what to keep in mind before you force push:
Confirm Your Changes: Always review your changes before force pushing, especially if you’re working on a project with others.
Collaborators: If you’re working on a project with others, it’s generally not a good idea to force push your changes unless absolutely necessary. Communicate with your team to avoid overwriting their work.
Backups: Before you force push, make sure you have a backup of your remote repository. This will allow you to recover your work if you accidentally delete important commits.
Remote Branch: Before force pushing, verify that you’re working on the correct branch. It’s easy to make a mistake and accidentally force push to the wrong branch.
Let me know if you have any more questions. I’m here to help!
See more new information: barkmanoil.com
Git Remove All Unpushed Commits: Clean Up Your Branch
Now, before we dive into the nitty-gritty, let’s understand what we’re dealing with here. Think of git as a time machine for your code. Each commit is like a snapshot of your project at a specific point in time. When you push your changes to a remote repository, you’re essentially sharing these snapshots with others.
So, if you’ve made a bunch of changes that you want to undo, you’re basically trying to erase those snapshots before they’re shared with the world. Git provides several ways to do this, but it’s crucial to proceed with caution. Once you remove a commit, it’s gone for good!
Let’s break down the most common scenarios where you might want to remove unpushed commits:
You made a mistake: Maybe you accidentally deleted a crucial file, introduced a bug, or messed up a feature. You want to rewind your project to a point before the mistake happened.
You want to clean up your history: Your commit messages are a mess, you’ve got a bunch of experimental branches, or you just want to streamline your project’s history for clarity.
You’re working on a feature branch: You’re developing a new feature and don’t want your changes to be seen by others until it’s complete. You might want to remove all your work from the branch to start over.
The `git reset` Command
The `git reset` command is your go-to tool for rewriting history. It lets you move the HEAD pointer (which represents your current working branch) to a different commit, effectively discarding all changes that happened after that point.
Here’s how it works:
`git reset –hard
Explanation:
`git reset`: This tells git that you want to reset your branch.
`–hard`: This flag tells git to completely discard any changes that were made after the specified commit. This means your working directory and staging area will be updated to match the state of the chosen commit.
`
Important: The `–hard` flag is powerful, but it’s also dangerous. Once you use it, you’ll lose all changes made after the target commit. So, make sure you have a backup of your project before using `git reset –hard`!
Example: Removing the Last Commit
Let’s say you’ve made a mistake in your latest commit and want to undo it. You can use the following command to reset your branch to the previous commit:
“`bash
git reset –hard HEAD^
“`
Explanation:
`HEAD^` represents the parent of the current commit, or the previous commit in the history.
This command will move your HEAD pointer to the previous commit and revert all changes that were made in the last commit.
Example: Removing Multiple Commits
If you want to remove multiple commits, you can specify a specific commit hash. For example, if you want to reset your branch to the commit that’s 5 commits behind the current HEAD, you can use this command:
“`bash
git reset –hard HEAD~5
“`
Explanation:
`HEAD~5` refers to the commit that’s 5 commits behind the current HEAD.
Alternatives to `git reset –hard`
While `git reset –hard` is a powerful tool, it’s not the only option for removing unpushed commits. Here are some other methods you can consider:
`git revert`: This command creates a new commit that undoes the changes introduced by the specified commit. This is a safer alternative to `git reset –hard` because it leaves a record of the changes you’ve undone.
`git rebase`: This command allows you to rewrite your branch history interactively. You can use it to reorder, squash, or edit commits, effectively cleaning up your history. This approach is more complex than `git reset –hard`, but it offers greater flexibility and control.
When Should You Use Each Method?
Here’s a breakdown of when to use each method for removing unpushed commits:
`git reset –hard`: Use this method when you want to completely discard changes and don’t need to keep a record of the removed commits. It’s a good option for fixing simple mistakes or cleaning up your branch history.
`git revert`: Use this method when you want to undo specific commits while preserving a record of the changes that were made. It’s a safer alternative to `git reset –hard` and is useful for reverting accidental changes or fixing bugs.
`git rebase`: Use this method when you need to perform complex changes to your branch history, such as reordering, squashing, or editing commits. It offers the most flexibility but also requires the most expertise.
Important Considerations
Before you start removing unpushed commits, consider these points:
Backup your project: Always make sure you have a backup of your project before you make any major changes to your git history.
Understand the risks: Be aware of the potential consequences of using `git reset –hard`. Once you remove commits, they’re gone for good.
Communicate with collaborators: If you’re working on a project with others, make sure you communicate with them before making any major changes to the shared history. This helps avoid confusion and ensures everyone is on the same page.
FAQs
Q: Can I remove unpushed commits after I’ve already pushed them to a remote repository?
A: Yes, but it’s more complicated. If you’ve already pushed your changes to a remote repository, you’ll need to force-push your changes to overwrite the remote history. This is generally not recommended because it can cause problems for other collaborators. If you need to rewrite history after pushing, make sure you understand the risks and communicate with your team.
Q: What if I want to undo a `git reset –hard`?
A: Unfortunately, there’s no way to undo a `git reset –hard` once it’s been performed. However, you can try to recover the lost commits using tools like `git reflog` or `git fsck`.
Q: What are some good practices for managing my git history?
A: Here are some tips:
Write descriptive commit messages: This makes it easier to understand the changes made in each commit and to track down issues.
Rebase your branches regularly: This helps to keep your branch history clean and manageable.
Use branches for feature development: This isolates your work and makes it easier to merge changes back into the main branch.
Don’t be afraid to experiment:Git is a powerful tool. Experiment with different commands and workflows to find what works best for you.
By following these tips and understanding the different ways to remove unpushed commits, you’ll be able to keep your git history clean and organized, ensuring a smooth and collaborative development process.
How do I delete unpushed git commits? – Stack Overflow
Delete the most recent commit, keeping the work you’ve done: git reset –soft HEAD~1 Delete the most recent commit, destroying the work you’ve done: git reset –hard HEAD~1 Stack Overflow
How do I Delete Unpushed Git Commits? – GeeksforGeeks
If you want to completely remove unpushed commits from your local repository history, including any changes they introduced, you can use the git reset GeeksForGeeks
How to Remove Unpushed Commits in Git | Delft Stack
Use the git reset Command to Remove Unpushed Commits in Git. Whenever we want to commit changes to the project directory, we can commit the changes using the git add and git commit Delft Stack
How To Remove An Unpushed Commit In Git? – Tim Mouskhelichvili
To remove all unpushed commits in Git, use the git reset command: bash git reset –hard origin This article will show different ways to remove unpushed commits Tim Mouskhelichvili
How to delete a git commit – graphite.dev
Follow the Interactive rebase steps mentioned above to remove the commit locally. Force push the changes: git push origin
Git – Remove All Commits – Clear Git History (Local & Remote)
How to clear Git history of a repository by removing all commits both locally and remotely. ShellHacks
How can I delete a commit in Git? | Learn Version Control with Git
How can I delete a commit in Git? Git offers a couple of tools to delete (or undo) older commits. The first step, therefore, is to find out which tool is best for your scenario. The Tower Git Client
Delete commits from a Git branch | Techie Delight
Delete commits from a Git branch. This post will discuss how to delete commits from a Git branch. 1. git reset. Here, the idea is to force reset the working directory to remove all commits which Techie Delight
Remove a git commit which has not been pushed – Stack Overflow
1 – Undo commit and keep all files staged: git reset –soft HEAD~ 2 – Undo commit and unstage all files: git reset HEAD~ 3 – Undo the commit and completely remove all stackoverflow.com
On undoing, fixing, or removing commits in git – GitHub Pages
To remove the last commit from git, you can simply run git reset –hard HEAD^ If you are removing multiple commits from the top, you can run git reset –hard HEAD~2 to remove sethrobertson.github.io
How Do I Undo The Most Recent Local Commits In Git
How To Undo / Revert A Local Commit In Git
Keep Your Git History Clean: Remove Unpushed Commits In Visual Studio
Delete Commits And Edit Commits With Git Rebase. Crazy Simple And Useful
How To Delete All Past Commits In A Git Branch (Short And Sweet!)
How Do I Discard Unstaged Changes In Git?
Link to this article: git remove all unpushed commits.
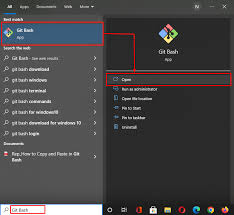
See more articles in the same category here: https://barkmanoil.com/bio/