Are you looking for an answer to the topic “python swap list elements“? We answer all your questions at the website barkmanoil.com in category: Newly updated financial and investment news for you. You will find the answer right below.
Keep Reading

Can you swap elements in a list Python?
Swap elements by value in a list. Use list. index(value) with each element as value to get their indices. Use multiple assignment to swap the value at each index in the list.
How do you switch multiple items in a list in Python?
For example, swapping the first and last elements in [“a”, “b”, “c”] results in [“c”, “b”, “a”]. Use list[index] to access the element at a certain index of a list. Use multiple assignment in the format val_1, val_2 = val_2, val_1 to swap the value at each index in the list.
Python Programming 22 – How to Swap Variables and List Elements
Images related to the topicPython Programming 22 – How to Swap Variables and List Elements

How do you swap values in Python?
The simplest way to swap the values of two variables is using a temp variable. The temp variables is used to store the value of the fist variable ( temp = a ). This allows you to swap the value of the two variables ( a = b ) and then assign the value of temp to the second variable.
Is there a swap function in Python?
A simple swap function in Python is generally considered a function that takes two initialized variables, a = val_a and b = val_b , and returns a result of a = val_b and b = val_a . The function accepts variables of arbitrary types, or more precisely, variables that are assigned with objects of arbitrary types.
How do you interchange elements in a list?
Store the element at pos1 and pos2 as a pair in a tuple variable, say get. Unpack those elements with pos2 and pos1 positions in that list. Now, both the positions in that list are swapped.
How do you swap two elements in an array?
The built-in swap() function can swap two values in an array . template <class T> void swap (T& a, T& b); The swap() function takes two arguments of any data type, i.e., the two values that need to be swapped.
How do you interchange the first and last element of a list in Python?
- Take the number of elements in the list and store it in a variable.
- Accept the values into the list using a for loop and insert them into the list.
- Using a temporary variable, switch the first and last element in the list.
- Print the newly formed list.
- Exit.
See some more details on the topic python swap list elements here:
Python program to swap two elements in a list – GeeksforGeeks
Given a list in Python and provided the positions of the elements, write a program to swap the two elements in the list. Examples:
How to swap elements in a list in Python – Adam Smith
Swap elements by index in a list. Use list[index] to access the element at a certain index of a list. Use multiple assignment in the format …
Python Program to Swap Two Elements in a List – Studytonight
In this tutorial, we will learn, how to swap two elements at given positions in a List. Swapping means exchanging the values of the two numbers.
How to Swap List Elements in Python? – Finxter
To swap two list elements x and y by value, get the index of their first occurrences using the list.index(x) and list.index(y) methods and assign the result to …
How do you switch two elements in a string in Python?
- s := make a list from the characters of s.
- for i in range 0 to size of s – 1, increase by 2, do. swap s[i], s[i+1] with s[i+1], s[i]
- join characters from s to make whole string and return.
How do you reverse a list in a for loop Python?
Iterate over the list using for loop and reversed() reversed() function returns an iterator to accesses the given list in the reverse order. Let’s iterate over that reversed sequence using for loop i.e. It will print the wordList in reversed order.
How do you swap variables?
The bitwise XOR operator can be used to swap two variables. The XOR of two numbers x and y returns a number that has all the bits as 1 wherever bits of x and y differ. For example, XOR of 10 (In Binary 1010) and 5 (In Binary 0101) is 1111 and XOR of 7 (0111) and 5 (0101) is (0010).
How do you swap two values?
- #include<stdio.h>
- int main()
- {
- int a=10, b=20;
- printf(“Before swap a=%d b=%d”,a,b);
- a=a+b;//a=30 (10+20)
- b=a-b;//b=10 (30-20)
- a=a-b;//a=20 (30-10)
How do you create a swap function in Python?
- P = int( input(“Please enter value for P: “))
- Q = int( input(“Please enter value for Q: “))
- # To swap the value of two variables.
- # we will user third variable which is a temporary variable.
- temp_1 = P.
- P = Q.
- Q = temp_1.
- print (“The Value of P after swapping: “, P)
Frequently Asked Python Program 9: How To Swap Any 2 Elements of a List
Images related to the topicFrequently Asked Python Program 9: How To Swap Any 2 Elements of a List

What does swap () mean in Python?
Python: swapping two variables
Swapping two variables refers to mutually exchanging the values of the variables. Generally, this is done with the data in memory. The simplest method to swap two variables is to use a third temporary variable : define swap(a, b) temp := a a := b b := temp.
What is the syntax of swap ()?
swap() function in C++
Here is the syntax of swap() in C++ language, void swap(int variable_name1, int variable_name2); If we assign the values to variables or pass user-defined values, it will swap the values of variables but the value of variables will remain same at the actual place.
How do you switch two elements in a tuple in Python?
- a=int(input(“Enter the first number :”))
- b=int(input(“Enter the second number :”))
- print (“a=”,a, “b=”,b)
- (a,b)=(b,a)
- print(“a=”,a, “b=”,b)
How would you reverse a Python list?
Python lists can be reversed in-place with the list. reverse() method. This is a great option to reverse the order of a list (or any mutable sequence) in Python. It modifies the original container in-place which means no additional memory is required.
How do you join elements in a list in Python?
If you want to concatenate a list of numbers into a single string, apply the str() function to each element in the list comprehension to convert numbers to strings, then concatenate them with join() .
How do you reverse an array in Python?
- Using reverse() Method. Similar to lists, the reverse() method can also be used to directly reverse an array in Python of the Array module. …
- Using reversed() Method. Again, the reversed() method when passed with an array, returns an iterable with elements in reverse order.
How do you swap places in an array?
- Create a temp variable and assign the value of the original position to it.
- Now, assign the value in the new position to original position.
- Finally, assign the value in the temp to the new position.
How do you swap without using a third variable?
- STEP 1: START.
- STEP 2: ENTER x, y.
- STEP 3: PRINT x, y.
- STEP 4: x = x + y.
- STEP 5: y= x – y.
- STEP 6: x =x – y.
- STEP 7: PRINT x, y.
- STEP 8: END.
Can you use array Destructuring to swap elements in an array?
Destructuring works great if you want to access object properties and array items. On top of the basic usage, array destructuring is convinient to swap variables, access array items, perform some immutable operations.
What is the difference between pop () function and Del statement of list?
pop() returns deleted value. The del keyword can delete the single value from a list or delete the whole list at a time. At a time it deletes only one value from the list. At a time it deletes only one value from the list.
Swapping elements in list – Python Programming
Images related to the topicSwapping elements in list – Python Programming
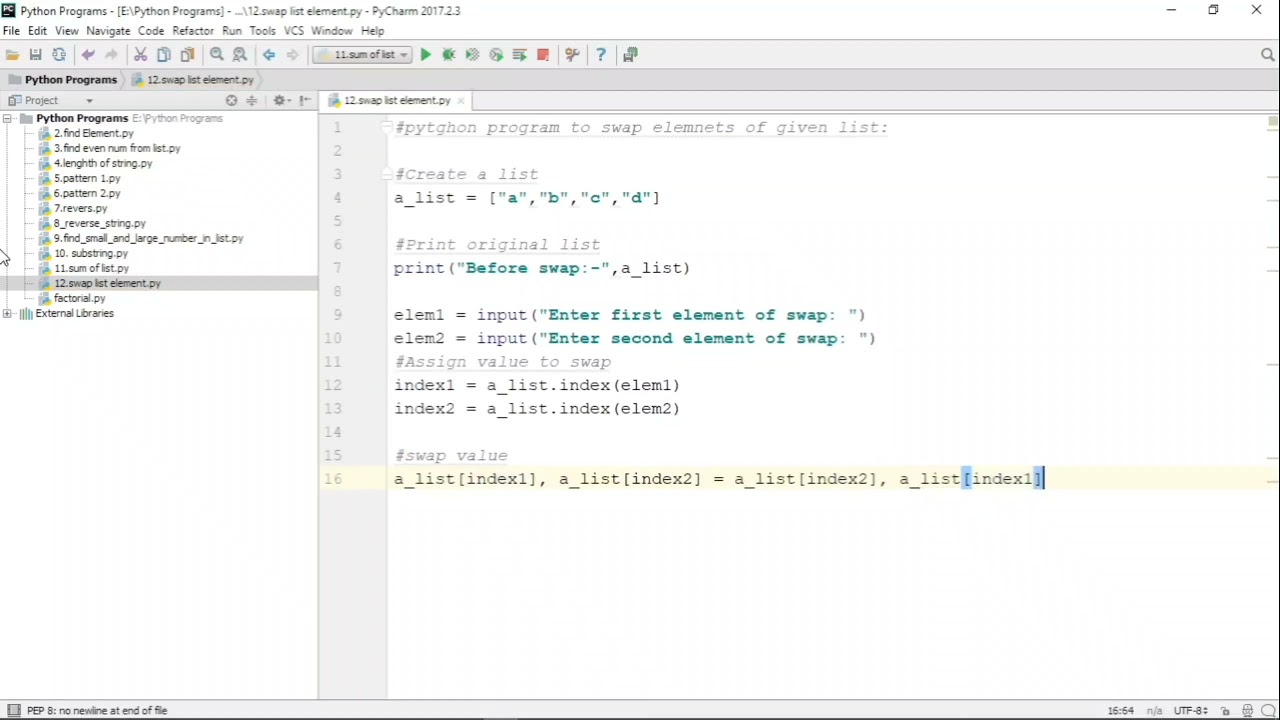
How do you sort a list without sorting in Python?
- number=[1,5,6,9,0]
- for i in range(len(number)):
- for j in range(i+1,len(number)):
- if number[i]<number[j]:
- number[i],number[j]=number[j],number[i]
- print(number)
How do global variables work in Python?
- ❮ Previous Next ❯
- Create a variable outside of a function, and use it inside the function. …
- Create a variable inside a function, with the same name as the global variable. …
- If you use the global keyword, the variable belongs to the global scope:
Related searches to python swap list elements
- check element in array python
- remove element in list python
- python – swap elements in string list
- python swap every two elements in list
- swap in python
- Python swap function
- swap elements in linked list python
- Remove element in list Python
- Move element in list python
- write a python program to swap the first and last element in the list
- python swap 2 list
- Swap in Python
- Swap in string Python
- how to swap the first and last elements in a list python
- swap all elements in list python
- python swap function
- write a function swap that swaps the first and last elements of a list argument. python
- delete element in list
- swap adjacent elements in list python
- move element in list python
- Python swap 2 list
- python 3 swap list elements
- swap in string python
- python swap list elements in place
Information related to the topic python swap list elements
Here are the search results of the thread python swap list elements from Bing. You can read more if you want.
You have just come across an article on the topic python swap list elements. If you found this article useful, please share it. Thank you very much.