Are you looking for an answer to the topic “python random float range“? We answer all your questions at the website barkmanoil.com in category: Newly updated financial and investment news for you. You will find the answer right below.
The random. uniform() function returns a random floating-point number between a given range in Python. For example, It can generate a random float number between 10 to 100 Or from 50.50 to 75.5.A range of floats is a list of numbers from start to stop incremented with a specified step interval. Ranges are typically “open”, meaning the value stop is omitted from the series. For example, a range from 1.0 to 3.0 with a step of 0.5 is [1.0, 1.5, 2.0, 2.5] .The Python range() works only with integers. It doesn’t support the float type, i.e., we cannot use floating-point/decimal value in any of its arguments. For example, If you use range() with float step argument, you will get a TypeError ‘float’ object cannot be interpreted as an integer .
- import random n = random. random() print(n)
- import random n = random. randint(0,22) print(n)
- import random randomlist = [] for i in range(0,5): n = random. randint(1,30) randomlist. …
- import random #Generate 5 random numbers between 10 and 30 randomlist = random.
Function | Description |
---|---|
random.randrange(-50, -5) | Returns any random negative integer between -50 to -6. |
random.sample(range(0, 1000), 10) | Returns a list of random numbers |
secrets.randbelow(10) | Returns a secure random number |

What is the range of random random in Python?
Function | Description |
---|---|
random.randrange(-50, -5) | Returns any random negative integer between -50 to -6. |
random.sample(range(0, 1000), 10) | Returns a list of random numbers |
secrets.randbelow(10) | Returns a secure random number |
What is the range of float in Python?
A range of floats is a list of numbers from start to stop incremented with a specified step interval. Ranges are typically “open”, meaning the value stop is omitted from the series. For example, a range from 1.0 to 3.0 with a step of 0.5 is [1.0, 1.5, 2.0, 2.5] .
Generate random float numbers in Python 3 | English
Images related to the topicGenerate random float numbers in Python 3 | English

Can Python range use floats?
The Python range() works only with integers. It doesn’t support the float type, i.e., we cannot use floating-point/decimal value in any of its arguments. For example, If you use range() with float step argument, you will get a TypeError ‘float’ object cannot be interpreted as an integer .
How do you generate random numbers in a specific range in Python?
- import random n = random. random() print(n)
- import random n = random. randint(0,22) print(n)
- import random randomlist = [] for i in range(0,5): n = random. randint(1,30) randomlist. …
- import random #Generate 5 random numbers between 10 and 30 randomlist = random.
How do you generate a random float in Python?
- Using random.uniform() function. You can use the random.uniform(a, b) function to generate a pseudorandom floating-point number n such that a <= n <= b for a <= b . …
- Using random. random() function. …
- Using random.randint() function. …
- Using numpy. …
- Using numpy.
What is difference between random () and Randint () function?
difference between random () and randint()
The random command will generate a rondom value present in a given list/dictionary. And randint command will generate a random integer value from the given list/dictionary.
How do you find the range of a float?
The decimal equivalent of a floating point number can be calculated using the following formula: Number = ( − 1 ) s 2 e − 127 1 ⋅ f , where s = 0 for positive numbers, 1 for negative numbers, e = exponent ( between 0 and 255 ) , and f = mantissa .
See some more details on the topic python random float range here:
Python Generate Random Float Number – Linux Hint
In Python, the random.uniform() function gives a random floating-point number, and that is within a specified range. For example, it can produce a random float …
Generate a random float in Python | Techie Delight
1. Using random.uniform() function. You can use the random. · 2. Using random.random() function. If you need to generate a random floating-point number in the …
How to generate a random float within a range in Python
Floats randomly generated within a range are between or equal to the endpoints of the range. For example, a randomly generated float within the range from 1.0 …
random — Generate pseudo-random numbers — Python 3.10 …
Almost all module functions depend on the basic function random() , which generates a random float uniformly in the semi-open range [0.0, 1.0). Python uses …
How do you make a float range?
NumPy’s arange() Function to Create a Range of Floats
Another option to produce a range of floats is to use the NumPy module’s arange() function. Where: start is the starting value of the range. stop specifies the end of the range.
What is the precision of float in Python?
Python Decimal default precision
The Decimal has a default precision of 28 places, while the float has 18 places.
How do you create a range interval in Python?
- Pass start and stop values to range() For example, range(0, 6) . Here, start=0 and stop = 6 . …
- Pass the step value to range() The step Specify the increment. …
- Use for loop to access each number. Use for loop to iterate and access a sequence of numbers returned by a range() .
How do you iterate through a list float in Python?
Use the for Loop to Convert All Items in a List to Float in Python. We can use the for loop to iterate through the list and convert each element to float type using the float() function. We can then add each element to a new list using the append() function.
How do you find the max float value in Python?
Max float Value With the sys.
In Python, we can use the sys. maxint function inside the built-in sys module to find out the maximum value that can be stored in an integer variable. We can also use the same sys module to find out the maximum value that can be stored inside a float variable with the sys. float_info .
How do you generate a random number in range 1 100?
The function to use is sample() which shuffles the input list, in the example below it shuffles the created list range(1,101) . That is to say, range(1,101) creates a list of numbers 1 to 100. Then the function sample() shuffles that list in random order.
How do you generate a random number between 1 and 10 in Python?
- Using the random.randint() function.
- Using the random.randrange() function.
- Using the random.sample() function.
- Using the random.uniform() function.
- Using the numpy.random.randint() function.
- Using the numpy.random.uniform() function.
- Using the numpy.random.choice() function.
Generate random float numbers in Python 3
Images related to the topicGenerate random float numbers in Python 3
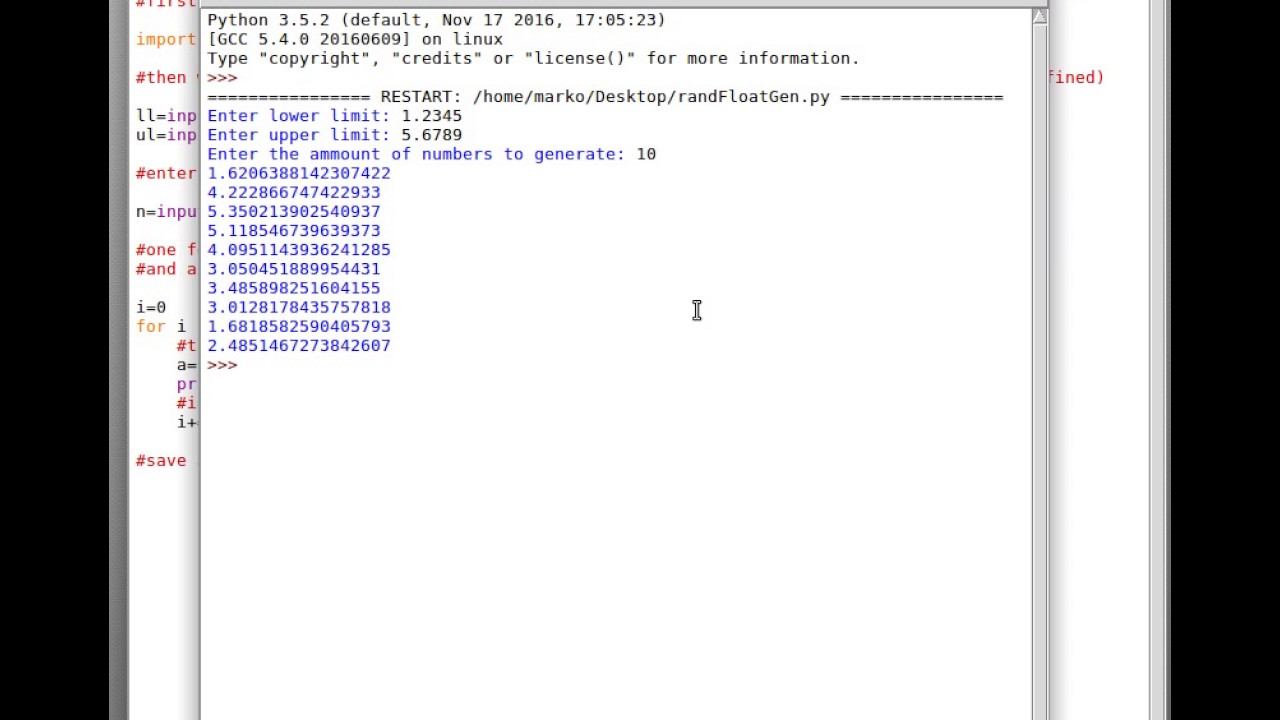
How can we generate a random number from a specified range in scratch?
Random Numbers
Scratch provides the pick random block specifically for this purpose. The block will randomly pick a number between and including the numbers used. The default settings will produce a random number between 1-10. The random number generator is found in the operator category of the blocks palette.
How do you round to 2 decimal places in python?
Python’s round() function requires two arguments. First is the number to be rounded. Second argument decides the number of decimal places to which it is rounded. To round the number to 2 decimals, give second argument as 2.
How do I limit decimal places in python?
format() to limit the string representation of a float to two decimal places. Call str. format(*args) with “{:. 2f}” as str and a float as *args to limit the float to two decimal places as a string.
How do I randomly select a list in python?
Use the random. sample() function when you want to choose multiple random items from a list without repetition or duplicates. There is a difference between choice() and choices() . The choices() was added in Python 3.6 to choose n elements from the list randomly, but this function can repeat items.
What is random () in Python?
random() function generate random floating numbers between 0 and 1. Syntax : random.random() Parameters : This method does not accept any parameter. Returns : This method returns a random floating number between 0 and 1.
How does random Randint work in Python?
Basically, the randint() method in Python returns a random integer value between the two lower and higher limits (including both limits) provided as two parameters. It should be noted that this method is only capable of generating integer-type random value.
What is the difference between Randrange () and Randint () Define with example?
The only differences between randrange and randint that I know of are that with randrange([start], stop[, step]) you can pass a step argument and random. randrange(0, 1) will not consider the last item, while randint(0, 1) returns a choice inclusive of the last item.
How do you find the range of data type?
- Formula. 2^(n-1) is the formula to find the maximum of an INT data type. In the preceding formula N (Size in bits) is the size of data type. …
- Output. 32 bits.
- Determine the maximum range of int. The formula is: 2^(n-1) here N=32.
How do you find the largest floating-point value?
Largest and Smallest Positive Floating Point Numbers:
Largest Number = (2 – 2–23)×2127 3.403 × 1038. If the result of a computation exceeds the largest number that can be stored in the computer, then it is called an overflow. Significand = 1.0. Exponent = 1 – 127 = –126.
How do you find the range of a floating-point in C?
To explicitly answer your question, that means for a 32 bit float, the largest value is (-1)^0 * 1.99999988079071044921875 * 2^128 , which is 6.8056469327705771962340836696903385088 × 10^38 according to Wolfram. The smallest value is the negative of that.
What does random random do in Python?
random() function generate random floating numbers between 0 and 1. Parameters : This method does not accept any parameter. Returns : This method returns a random floating number between 0 and 1.
How do you generate random 50 numbers in Python?
The randint() method to generates a whole number (integer). You can use randint(0,50) to generate a random number between 0 and 50. To generate random integers between 0 and 9, you can use the function randrange(min,max) .
How to get a random number between a float range in python
Images related to the topicHow to get a random number between a float range in python
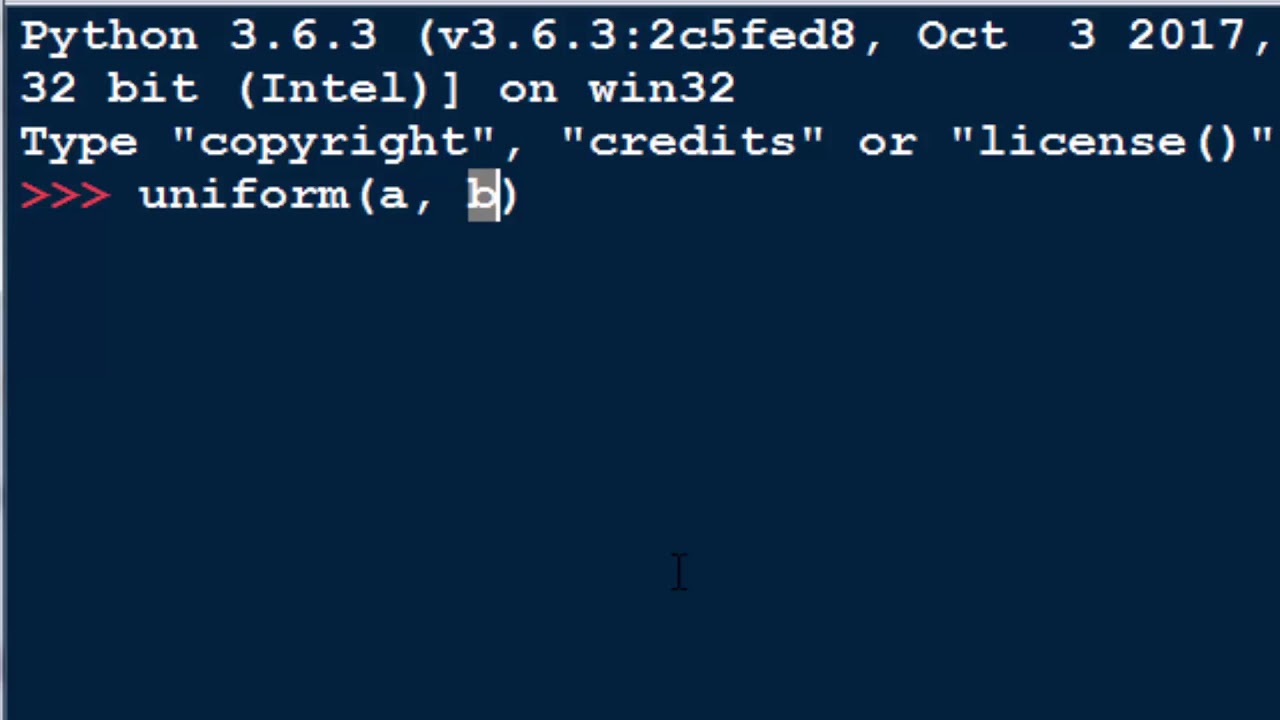
What is random Randint in Python?
Python Random randint() Method
The randint() method returns an integer number selected element from the specified range. Note: This method is an alias for randrange(start, stop+1) .
What is random seed in Python?
Python Random seed() Method
The seed() method is used to initialize the random number generator. The random number generator needs a number to start with (a seed value), to be able to generate a random number. By default the random number generator uses the current system time.
Related searches to python random float range
- random float c
- random float number python
- Random 0, 1 Python
- python random float example
- python numpy random float range
- random 0 1 python
- python get random float between range
- Random float number Python
- random list of floats python
- Use random in Python
- python generate random float numbers in range
- Random float C++
- Random float Python
- use random in python
- python random list of floats
- python random float between range
- Numpy random in range
- get random float value in range python
- random float python
- random number python
- python generate random float in range
- python random float number between range
- python3 random float range
- numpy random in range
Information related to the topic python random float range
Here are the search results of the thread python random float range from Bing. You can read more if you want.
You have just come across an article on the topic python random float range. If you found this article useful, please share it. Thank you very much.